PROJECT: Infinity Book
Welcome to my portfolio page for CS2103T AY 2017/2018 Sem 2 project - Infinity Book.
1. Overview
Infinity Book is a desktop application that provides Tech recruiters end-to-end support, from searching for candidates,to adding job postings and saving their resumes and interviews.
2. Summary of contributions
Code contributed: [Functional code] [Test code]_
2.1. Major enhancement: added the interview management.
-
Motivation: Interviewing is one of the important steps in recruiting process. HR may need a tool to manage interview efficiently.
-
What it does: allows the user to add the interview relevant command which are add interview command, find interview command, listing interview command.
-
Highlights: This enhancement affects existing commands and commands to be added in future. It required an in-depth analysis of design alternatives. The implementation too was challenging as it required changes to existing model, ui, and storage.
2.2. Minor enhancement: added the view command.
-
Motivation: The HR may want to find more details information of specific candidates. The view command helps HR with this problem.
-
What it does: allows user to view a specific person by email ID and display the linked page of the person.
-
Highlights: This enhancement affects existing commands and commands to be added in future. It required an in-depth analysis of design alternatives. The implementation too was challenging as it required changes to existing commands.
2.3. Other contributions:
2.3.1. Project management:
-
Managed releases
v1.5rc
on GitHub -
Managed milestones on GitHub.
3. Contributions to the User Guide
3.1. Viewing a person: view
-
Description: viewing the person records and linking page locating by emailID
-
Format:
view EmailID
-
Examples:
view abcd@gmail.com
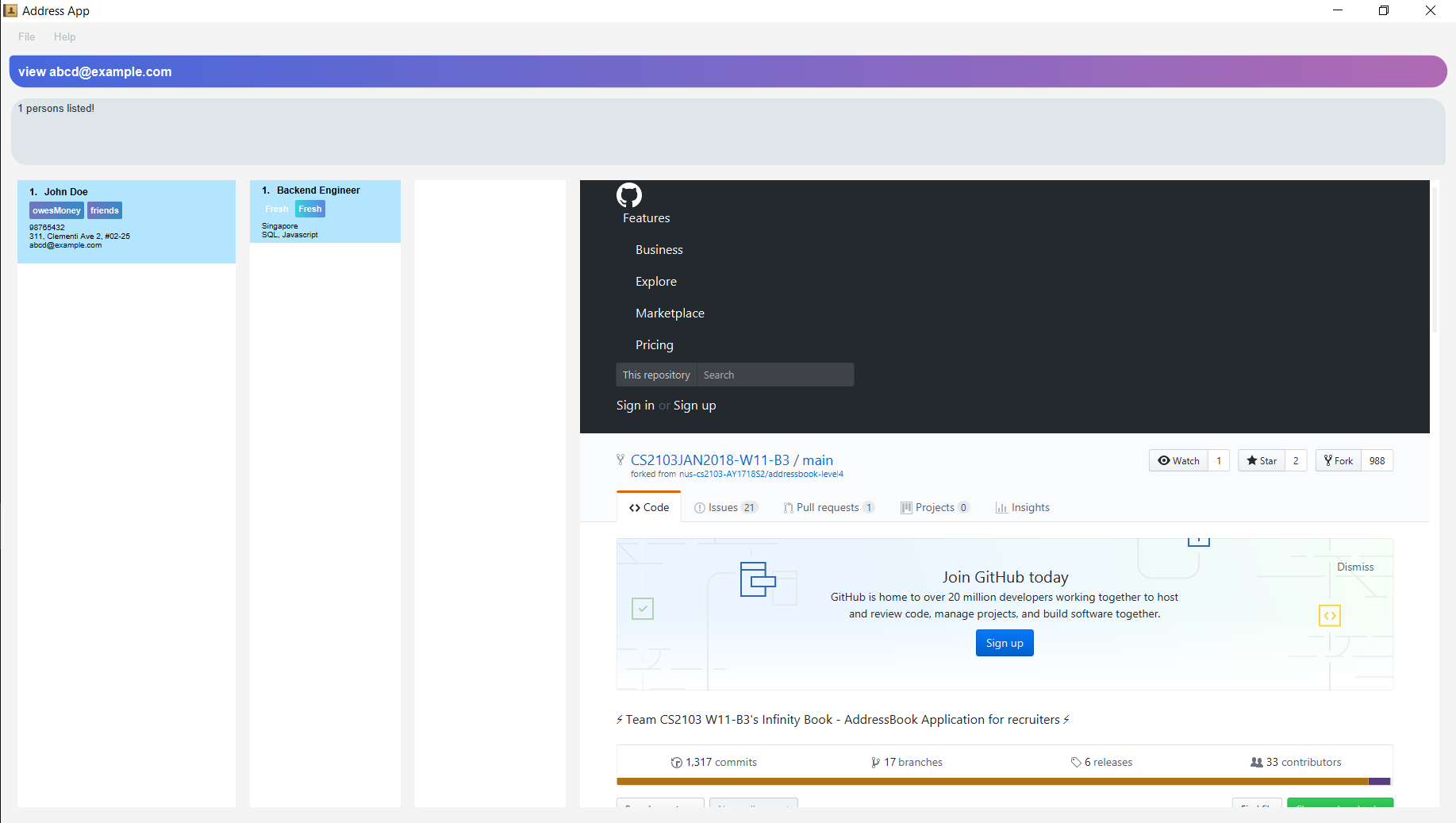
-
Returns the person whose email is
john@gmail.com
-
Displays all the person’s information and resume in the browser panel.
|
If there are two persons with same email, the viewcommand will render URL of the first person |
When a person has no linked page, it will display black board in the browser panel. |
3.2. Managing Interview
This section describes command available for managing interview in the Infinity Book.
3.2.1. Add Interview Command
-
Description: Add a Interview to Infinity Book.
-
Format:
addInterview i/Interview Title n/Interviewee l/LOCATION d/DATE
-
Examples:
addInterview i/SE Interview n/John l/One North d/30.1.2018
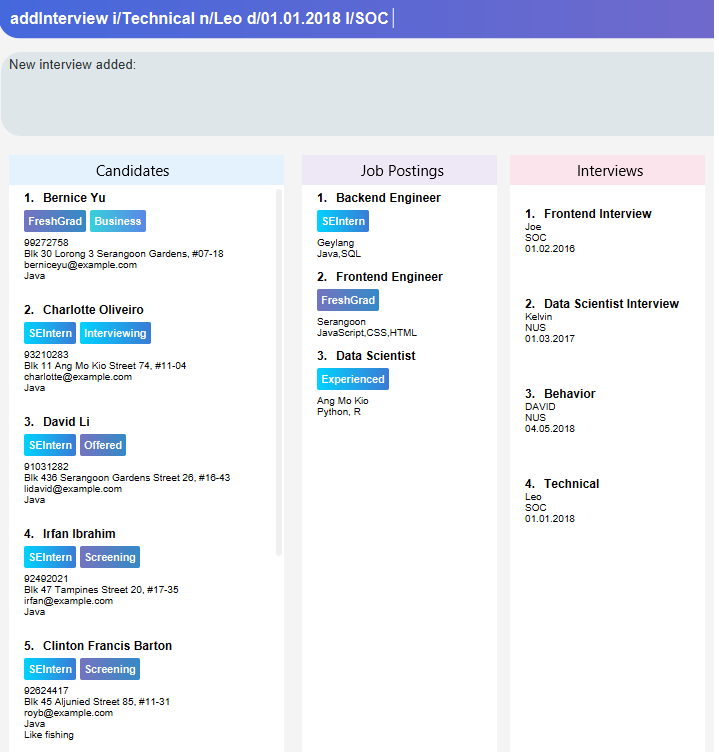
-
Add the interview with predicate name, location, and date
-
Displays the added interview in list of Interview panel.
3.2.2. List Interview
-
Description: List all interviews of Infinity Book.
-
Format:
listInterview
-
Example:
listInterview
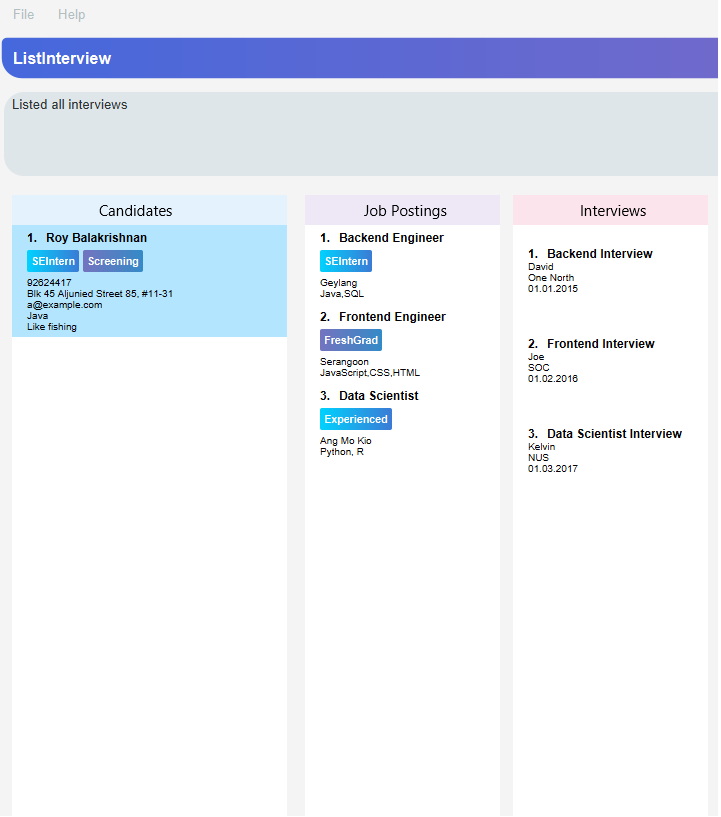
3.2.3. Delete Interview
-
Description: Remove an interview from Infinity Book using the index from latest listing.
-
Format:
deleteInterview INDEX
-
Examples:
deleteInterview 1
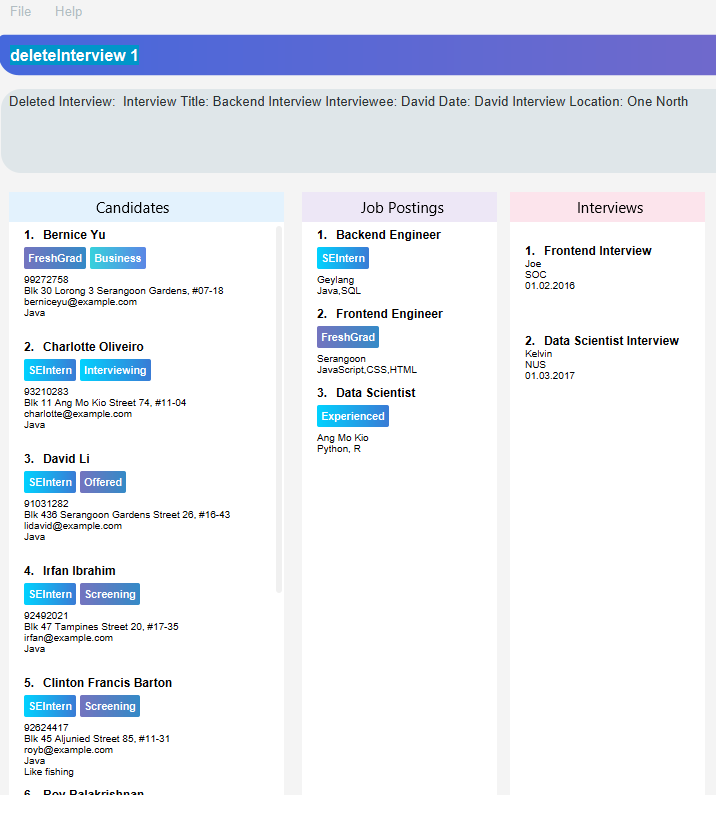
4. Contributions to the Developer Guide
4.1. Display relevant Github or resume page. (Since v1.3)
As a candidate may have linked online resume or github page. The recruiter may want to consider these pages.
4.1.1. Design Considerations
Aspect: Aspects: Implementation of removing/adding a linked page.
Alternative 1:(current choice) Each person has a page, which is resume or github.
Add the view command to view a specific person, and view the linked page on the browser panel.
Pros: The page can be linked to the person, and it is easier to view by person’s email
Cons: Need to modify current implementation of person model
Alternative 2: Add a new command to view specific page, which is hard code URL.
Pros: No need to modify current person
Cons: Hard to maintain the hard code URL
4.1.2. Implementation details
-
ViewCommandParser
class will extract emails ID from user input, form a predicate, then pass it toViewCommand
class. -
ViewCommand
will take in the predicate and update the list of Persons by email ID, and change the browser panel accordingly. source, java]
public class EmailFilter implements Predicate<Person> { private final String email; public EmailFilter (Email email) { this.email = email.toString(); } @Override public boolean test(Person person) { return person.getEmail().toString().equals(this.email); } @Override public boolean equals(Object other) { return other == this // short circuit if same object || (other instanceof EmailFilter // instanceof handles nulls && this.email.equals(((EmailFilter) other).email)); // state check } }
4.2. Interview Management [Since v1.5rc]
Interviewing is one of the important steps in recruiting process. HR may need a tool to manage interview efficiently. The Infinity Book will need interview model with basic features including adding interview, listing interview, and deleting interview.
-
Model Component:
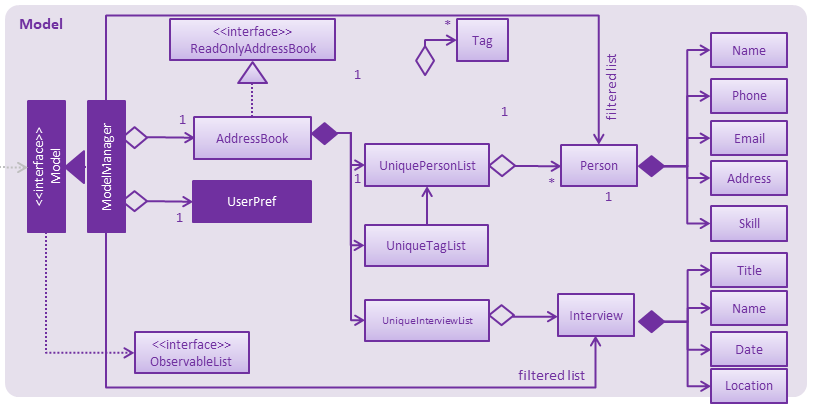
-
Storage Component:
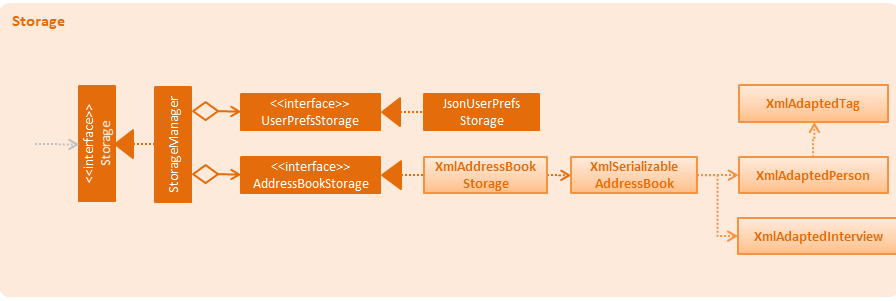
4.2.1. Adding an interview
The recruiter may want to conduct an interview with candidates, and maintain an interview lists.
Aspects: Implementation of removing/add interview with candidates.
Alternative 1: (current choice): Add a new model interview including many
sub fields such as Date, Location, List of Questions.
Pros: It is easier to implement other commands such as find Interview, delete
Interview, add questions.
Cons: It takes time to create new model.
Alternative 2: Add a new field interview to each candidates and maintain
according to each candidates.
Pros: It is easier to implement.
Cons: It is difficult to search specific interview effectively.
-
Current implementation details
-
AddInterviewCommandParser
class will extract interview title, interviewee name, date, and interview location from user input, form a new interview, then pass it toAddInterviewCommand
class. -
AddInterviewCommand
will take in the interview and call add new interview to storage in model management.
-
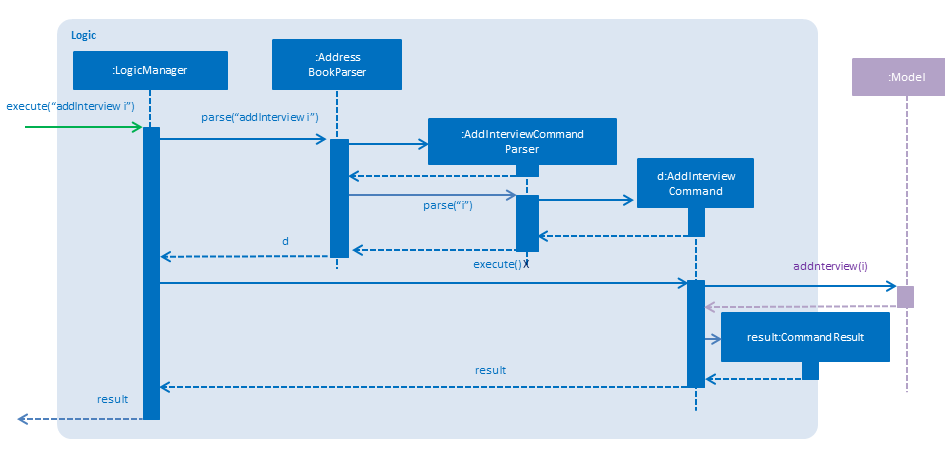
public synchronized void addInterview(Interview interview) throws DuplicateInterviewException {
addressBook.addInterview(interview);
updateFilteredInterviewList(PREDICATE_SHOW_ALL_INTERVIEWS);
indicateAddressBookChanged();
}
4.2.2. List interview
The recruiter may want to take a look at all of the interviews.
Aspects: Implementation of listing all the interviews.
Alternative 1: (current choice) create a new command to listing all the interview.
Pros: It is easier to use and modify.
Cons: New command is needed.
Alternative 2: each candidate has been linked to an interview, listing all the
interview when listing all candidates.
Pros: No need to create new command, just need to edit current list comamnd
Cons: It increases coupling and it is harder to implement.
-
Current implementation details
-
AddressBookParser
class will parse the commandlistInterview
from CLI and call ListInterviewCommand. -
ListInterviewCommand
will call updateFilteredList in model and list all the interviews in storage.
-
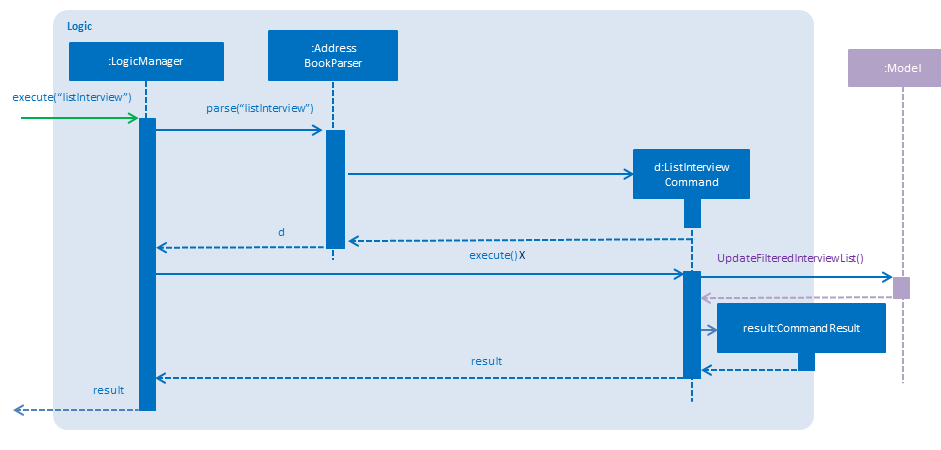
public void updateFilteredInterviewList(Predicate<Interview> predicate) {
requireNonNull(predicate);
filteredInterviews.setPredicate(predicate);
}
4.2.3. Deleting Interview
After conducted interview, the HR may want to delete the interview from the Infinity Book.
Aspects: Implementation of deleting an interview:
Alternative 1: (current choice) create deleting command for deleting interview by index
Pros: It can be easily to use follow the index of listing interview command
Cons: It requires users to use two commands
Alternative 2: create deleting command for deleting interview by name.
Pros: It requires addtional tools to match interview
Cons: It is easier for user to use.
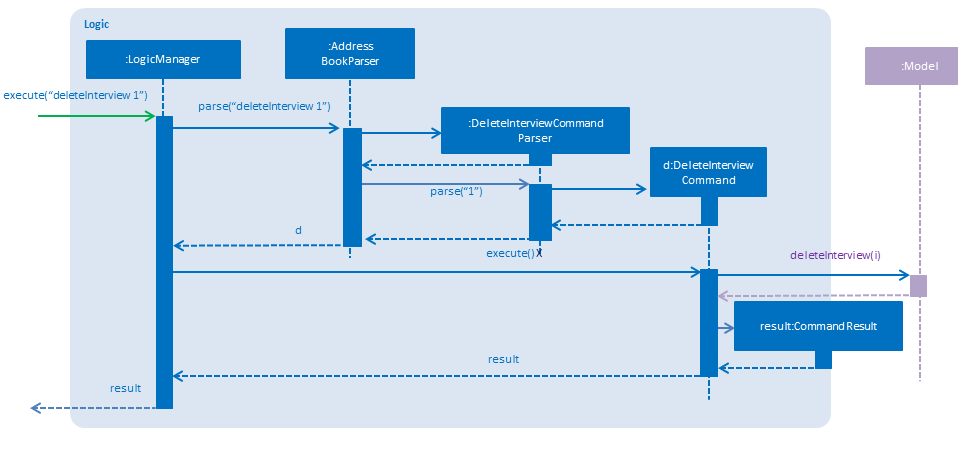
-
Current implementation details
-
DeletingInterviewParser
class will extract the index of the interview needed to be deleted and pass in toDeleteInterviewCommand
. -
DeleteInterviewCommand
will take in the index and update the interview list accordingly.
-
public synchronized void deleteInterview(Interview target) throws InterviewNotFoundException {
addressBook.removeInterview(target);
indicateAddressBookChanged();
}